CS615 – Software Engineering I
|
Lecture 2
|
Unified Process Model (Chapter 3, Pressman, 6th ed)
Unified Process—a “use-case driven, architecture-centric, iterative
and incremental” software process closely aligned with the Unified Modeling
Language (UML)

UP Phases
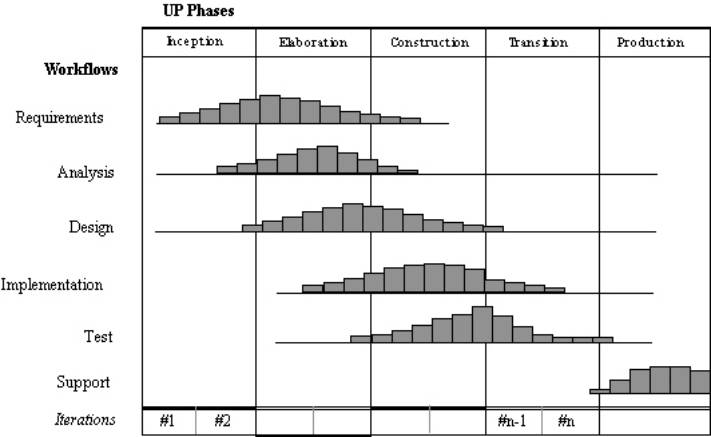
UP Work Products
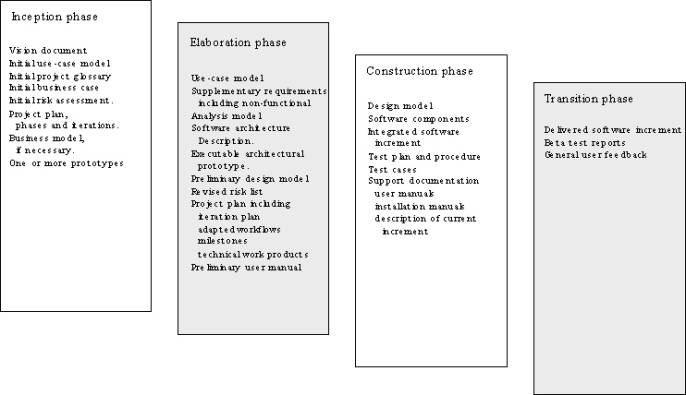
The Manifesto for Agile
Software Development
“We are uncovering better
ways of developing software by doing it and helping others do it. Through this work we have come to value:
- Individuals and interactions over processes and
tools
- Working software over comprehensive
documentation
- Customer collaboration over contract negotiation
- Responding to change over following a plan
That is, while there is
value in the items on the right, we value the items on the left more.” - Kent
Beck et al
What is “Agility”?
- Effective (rapid and adaptive) response to
change
- Effective communication among all stakeholders
- Drawing the customer onto the team
- Organizing a team so that it is in control of
the work performed
Yielding …
- Rapid, incremental delivery of software
An Agile Process
- Is driven by customer descriptions of what is
required (scenarios)
- Recognizes that plans are short-lived
- Develops software iteratively with a heavy
emphasis on construction activities
- Delivers multiple ‘software increments’
- Adapts as changes occur
Extreme Programming (XP)
The most widely used agile
process, originally proposed by Kent Beck
XP Planning
- Begins with the creation of “user stories”
- Agile team assesses each story and assigns a cost
- Stories are grouped to for a deliverable
increment
- A commitment is made on delivery date
- After the first increment “project velocity” is
used to help define subsequent delivery dates for other increments
XP Design
- Follows the KIS principle
- Encourage the use of CRC cards (see Chapter 8)
- For difficult design problems, suggests the
creation of “spike solutions”—a design prototype
- Encourages “refactoring”—an iterative refinement
of the internal program design
XP Coding
- Recommends the construction of a unit test for a
store before coding commences
- Encourages “pair programming”
XP Testing
- All unit tests are executed daily
- “Acceptance tests” are defined by the customer
and excuted to assess customer visible functionality
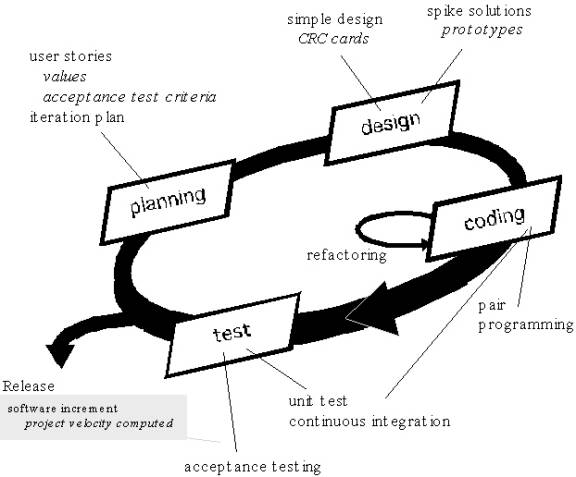
Adaptive Software Development
- Originally proposed by Jim Highsmith
- ASD — distinguishing features:
- Mission-driven planning
- Component-based focus
- Uses “time-boxing” (See Chapter 24)
- Explicit consideration of risks
- Emphasizes collaboration for requirements
gathering
- Emphasizes “learning” throughout the process
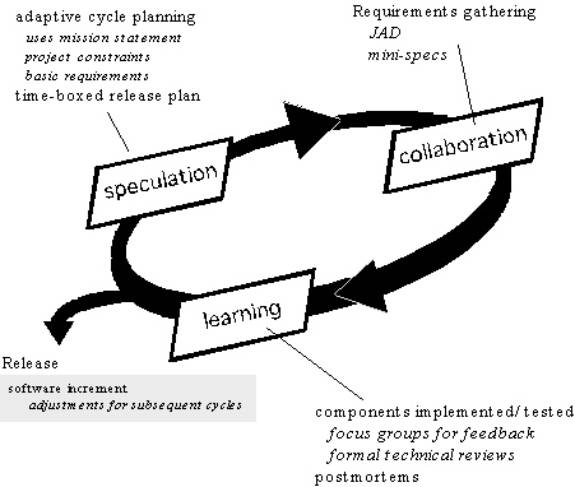
Dynamic Systems Development Method
Promoted by the DSDM
Consortium (http://www.dsdm.org/)
DSDM—distinguishing features
- Similar in most respects to XP and/or ASD
- Guiding principles:
- Active user involvement is imperative.
- DSDM teams must be empowered to make decisions.
- The focus is on frequent delivery of products.
- Fitness for business purpose is the essential
criterion for acceptance of deliverables.
- Iterative and incremental development is
necessary to converge on an accurate business solution.
- All changes during development are reversible.
- Requirements are baselined at a high level
- Testing is integrated throughout the life-cycle.
Scrum
Originally proposed by
Schwaber and Beedle
Distinguishing features
- Development work is partitioned into “packets”
- Testing and documentation are on-going as the
product is constructed
- Work occurs in “sprints” and is derived from a “backlog”
of existing requirements
- Meetings are very short and sometimes conducted
without chairs
- “demos” are delivered to the customer with the
time-box allocated
Crystal
Proposed by Cockburn and
Highsmith
Crystal—distinguishing
features
- A family of process models that allow “maneuverability”
based on problem characteristics
- Face-to-face communication is emphasized
- Suggests the use of “reflection workshops” to
review the work habits of the team
Feature Driven Development
Originally proposed by Peter
Coad et al (PDF)
FDD—distinguishing features
- Emphasis is on defining “features”
- a feature “is a client-valued function that can
be implemented in two weeks or less.”
- Uses a feature template
- <action> the <result> <by | for
| of | to> a(n) <object>
total the value of the sale
calculate the interest for the bank account
total the hours worked for the pilot
- A features list is created and “plan by feature”
is conducted
- Design and construction merge in FDD
Agile Modeling
Originally proposed by Scott
Ambler - http://www.agilemodeling.com/
Suggests a set of agile
modeling principles
- Model with a purpose
- Use multiple models
- Travel light
- Content is more important than representation
- Know the models and the tools you use to create
them
- Adapt locally
Chapter 5 - Practice: A Generic View
What is “Practice”?
- Practice is a broad array of concepts,
principles, methods, and tools that you must consider as software is
planned and developed.
- It represents the details—the technical considerations
and how to’s—that are below the surface of the software process—the things
that you’ll need to actually build high-quality computer software.
The Essence of Practice
- Understand the problem (communication and
analysis).
- Plan a solution (modeling and software design).
- Carry out the plan (code generation).
- Examine the result for accuracy (testing and
quality assurance)
Core Software Engineering Principles
- Provide value to the customer and the user
- KIS—keep it simple!
- Maintain the product and project “vision”
- What you produce, others will consume
- Be open to the future
- Plan ahead for reuse
- Think!
Software Engineering Practices
The generic process
framework
- Communication
- Planning
- Modeling
- Construction
- Deployment
Communication Practices
Principles
- Listen
- Prepare before you communicate
- Facilitate the communication
- Face-to-face is best
- Take notes and document decisions
- Collaborate with the customer
- Stay focused
- Draw pictures when things are unclear
- Move on …
- Negotiation works best when both parties win.
Initiation
- The parties should be physically close to one
another
- Make sure communication is interactive
- Create solid team “ecosystems”
- Use the right team structure
An abbreviated task set
- Identify who it is you need to speak with
- Define the best mechanism for communication
- Establish overall goals and objectives and
define the scope
- Get more detailed
- Have stakeholders define scenarios for usage
- Extract major functions/features
- Review the results with all stakeholders
Planning Practices
Principles
- Understand the project scope
- Involve the customer (and other stakeholders)
- Recognize that planning is iterative
- Estimate based on what you know
- Consider risk
- Be realistic
- Adjust granularity as you plan
- Define how quality will be achieved
- Define how you’ll accommodate changes
- Track what you’ve planned
Initiation
Questions to ask
- Why is the system begin developed?
- What will be done?
- When will it be accomplished?
- Who is responsible?
- Where are they located (organizationally)?
- How will the job be done technically and
managerially?
- How much of each resource is needed?
Planning Practices
Abbreviated task set
- Re-assess project scope
- Assess risks
- Evaluate functions/features
- Consider infrastructure functions/features
- Create a coarse granularity plan
- Number of software increments
- Overall schedule
- Delivery dates for increments
- Create fine granularity plan for first increment
- Track progress
Modeling Practices
We create models to gain a
better understanding of the actual entity to be built
- Analysis models represent the customer requirements by
depicting the software in three different domains: the information domain,
the functional domain, and the behavioral domain.
- Design models represent characteristics of the software that help practitioners
to construct it effectively: the architecture, the user interface, and
component-level detail.
Analysis Modeling Practices
Analysis modeling principles
- Represent the information domain
- Represent software functions
- Represent software behavior
- Partition these representations
- Move from essence toward implementation
- Elements of the analysis model (Chapter 8)
- Data model
- Flow model
- Class model
- Behavior model
n
Design Modeling Practices
Principles
- Design must be traceable to the analysis model
- Always consider architecture
- Focus on the design of data
- Interfaces (both user and internal) must be
designed
- Components should exhibit functional
independence
- Components should be loosely coupled
- Design representation should be easily
understood
- The design model should be developed iteratively
Elements of the design model
- Data design
- Architectural design
- Component design
- Interface design
Construction Practices
Preparation principles:
Before
you write one line of code, be sure you:
- Understand of the problem you’re trying to solve
(see communication and modeling)
- Understand basic design principles and concepts.
- Pick a programming language that meets the needs
of the software to be built and the environment in which it will operate.
- Select a programming environment that provides
tools that will make your work easier.
- Create a set of unit tests that will be applied
once the component you code is completed.
Coding principles:
As
you begin writing code, be sure you:
- Constrain your algorithms by following
structured programming [BOH00] practice.
- Select data structures that will meet the needs
of the design.
- Understand the software architecture and create
interfaces that are consistent with it.
- Keep conditional logic as simple as possible.
- Create nested loops in a way that makes them
easily testable.
- Select meaningful variable names and follow
other local coding standards.
- Write code that is self-documenting.
- Create a visual layout (e.g., indentation and
blank lines) that aids understanding.
Validation Principles:
After
you’ve completed your first coding pass, be sure you:
- Conduct a code walkthrough when appropriate.
- Perform unit tests and correct errors you’ve
uncovered.
- Refactor the code.
Testing Principles
·
All tests should be
traceable to requirements
- Tests should be planned
- Testing begins “in the small” and moves toward
“in the large”
- Exhaustive testing is not possible
Deployment Practices
Principles
- Manage customer expectations for each increment
- A complete delivery package should be assembled
and tested
- A support regime should be established
- Instructional materials must be provided to
end-users
- Buggy software should be fixed first, delivered
later